The fastest way to validate your Golang schemas
Zius Schema Validator
With custom messages and custom validation
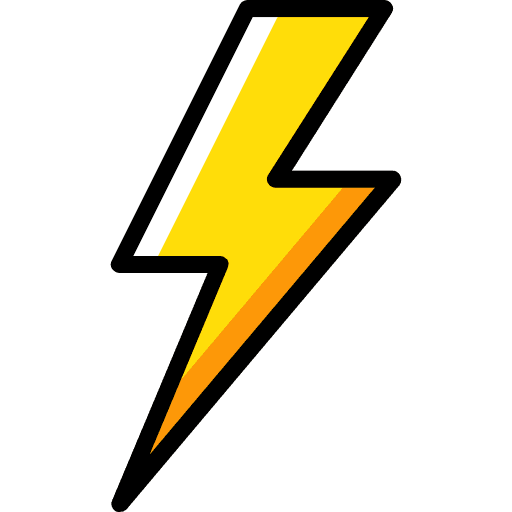
Getting Started
Install zius using Go Modules:
$go get github.com/vinibgoulart/zius
Import the zius in your project:
import ( zius "github.com/vinibgoulart/zius")
Define zius in your Struct:
type Simple struct { Name string `zius:"required:Name is required,string"`}
Start to validate your structs:
func main() { _, err := zius.Validate(Simple{ Name: "Vinicius" })}
Validations
Type | Description | Tag Value | Example |
---|---|---|---|
required | The field is required | false | required:Field is required |
int | The field must be an integer | false | int:Field must be an integer |
string | The field must be a string | false | string:Field must be a string |
number | The field must be a number | false | number:Field must be a number |
email | The field must be an email | false | email:Field must be an email |
phone | The field must be a phone number | false | phone:Field must be a phone number |
regex | The field must match the regex pattern | true | regex={^[a-zA-Z]\d{1,2}$}:Field must match the pattern |
equals | The field must be equal to another field | true | equals={BOY|GIRL}:Field must be equal to BOY or GIRL |
struct | The field must be a struct | false | struct:Field must be a struct |
array | The field must be an array | false | array:Field must be an array |
Custom Messages
Define custom messages for each field in your struct
type Simple struct { Name string `zius:"required:Name is required,string"` Age string `zius:"required:Age is required,int"`}
Handling Errors
You can handle the errors returned by the validation function, you can handle all errors at once or each one individually.
func main() { errors, err := zius.Validate(Simple{ Name: "Vinicius" })}
The Validate function returns an array of errors and an error, you can check if the error is not nil and handle it.
The error contains the following fields:
type Error struct { Struct string Field string Error error}
Examples
Check out some examples of how to use Zius our the repository. Zius Repository.
Feel free to contribute to the repository, suggest new features, or ask for help in the repository issues.